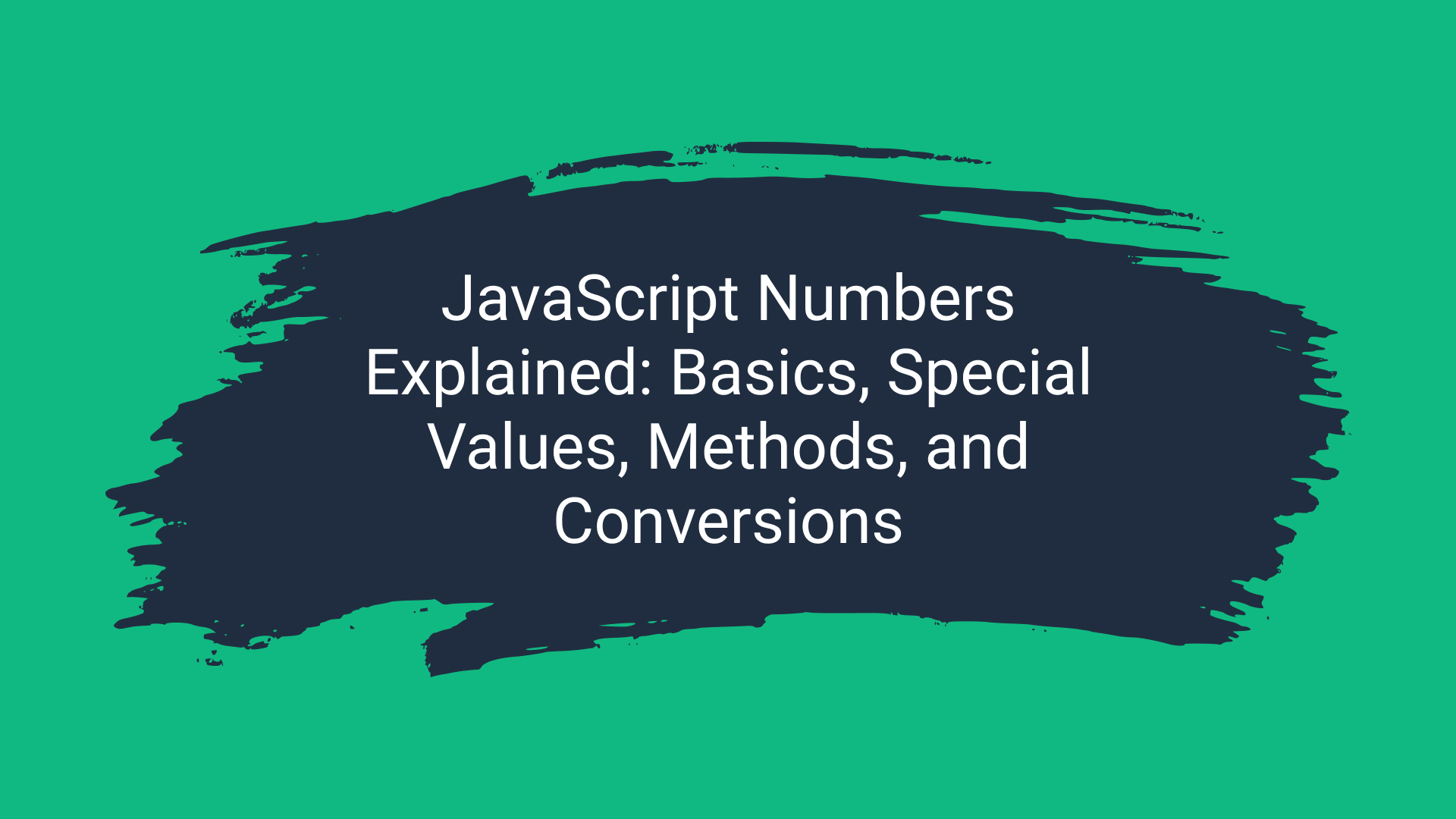
JavaScript Numbers Explained: Basics, Special Values, Methods, and Conversions
Overview
Numbers in JavaScript play a critical role in almost every application. Whether you are calculating values, generating random numbers, or converting data types, understanding JavaScript numbers and their related methods is essential. In this guide, we'll break down the basics of JavaScript numbers, special values, commonly used methods, and number conversion techniques in an easy-to-follow manner.
Understanding Basic Numbers
- Integer: Whole numbers without a decimal point.
Example:
let num1 = 10;
- Float: Numbers with a decimal point.
Example:
let num2 = 3.14;
Special Number Values
JavaScript has some special number values:
- Infinity: Represents positive infinity, e.g.,
Example:
let infinity = Infinity;
- Negative Infinity: Represents negative infinity, e.g.,
Example:
let negativeInfinity = -Infinity;
- NaN (Not-a-Number): Represents a non-numeric value, e.g.,
Example:
let notANumber = NaN;
Useful Number Methods
JavaScript offers built-in methods to manipulate numbers:
- Math.round(): Rounds a number to the nearest integer.
Example:
Math.round(3.14); // Output: 3
- Math.floor(): Rounds a number down to the nearest integer.
Example:
Math.floor(3.14); // Output: 3
- Math.ceil(): Rounds a number up to the nearest integer.
Example:
Math.ceil(3.14); // Output: 4
- Math.abs(): Returns the absolute value of a number.
Example:
Math.abs(-10); // Output: 10
Generating Random Numbers
- Math.random(): Generates a random number between 0 and 1.
Example:
Math.random(); // Output: 0.12345 (random)
- Random Integer in Range: Combine with Math.floor() to generate a random integer.
Example:
Math.floor(Math.random() * 100); // Output: A random number between 0 and 99
Converting Strings to Numbers
- Number(): Converts a string to a number.
Example:
Number("42"); // Output: 42
- parseInt(): Parses a string to an integer.
Example:
parseInt("42.5"); // Output: 42
- parseFloat(): Parses a string to a floating-point number.
Example:
parseFloat("3.14"); // Output: 3.14