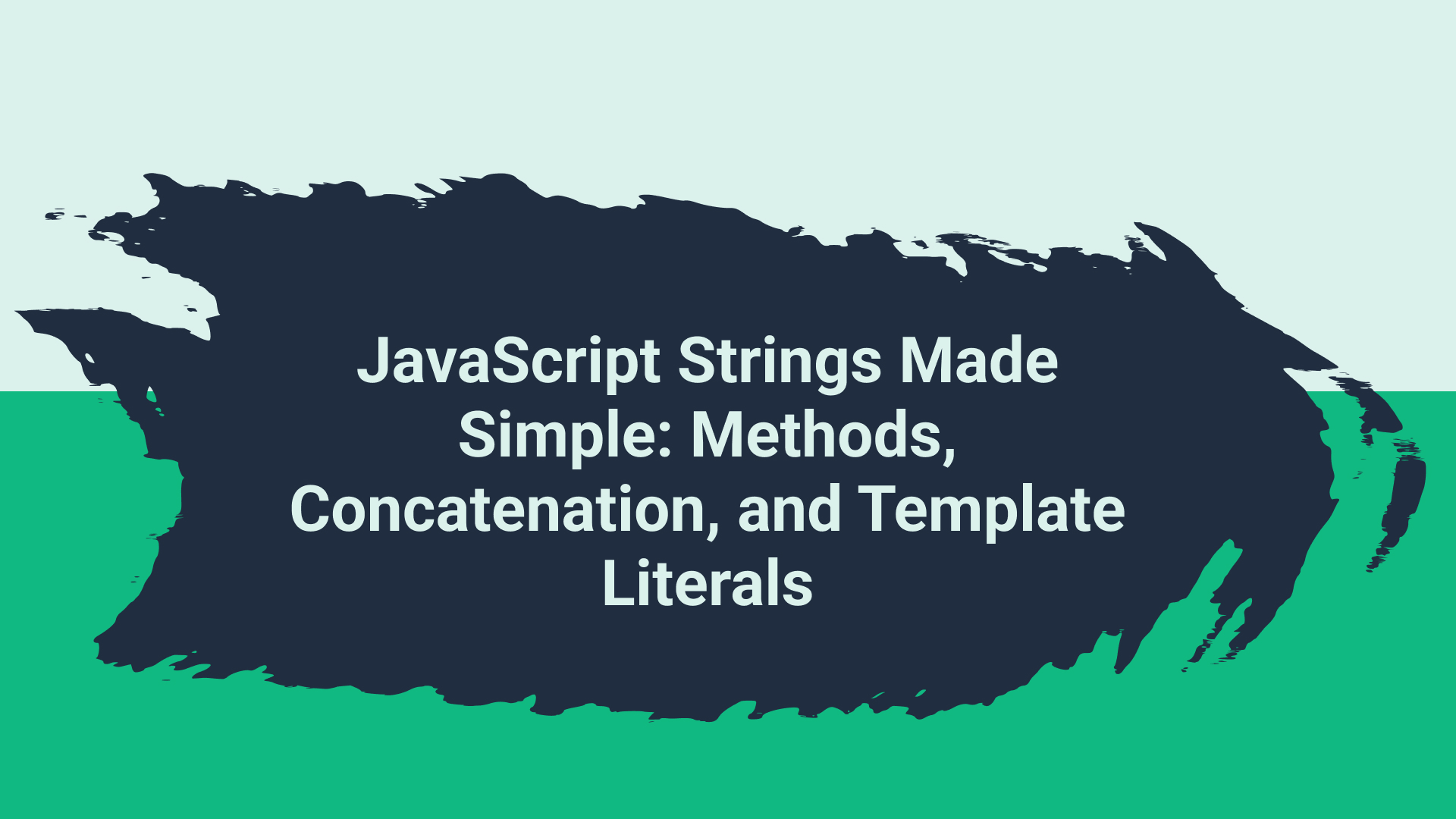
JavaScript Strings Made Simple: Methods, Concatenation, and Template Literals
Overview
Strings are a fundamental part of JavaScript and are used to handle and manipulate text. In this guide, we’ll explore string declarations, concatenation, and useful methods for modifying and extracting string data. With step-by-step examples, you’ll learn how to effectively work with strings in JavaScript and apply these concepts in your projects.
Introduction
Strings are one of the most commonly used data types in JavaScript. They represent text and can be manipulated in various ways. Whether you're creating user messages, formatting data, or building templates, strings are indispensable. Let’s dive into how to use them effectively.
String Declarations
There are three ways to declare strings in JavaScript:
- 1. Single Quotes:
Example:
let singleQuoteString = 'Hello, World!';
- 2. Double Quotes:
Example:
let doubleQuoteString = "Hello, World!";
- 3. Template Literals:
Template literals use backticks (`) and allow for embedded variables and multi-line strings.
Example:
let templateLiteralString = `Hello, World!`;
String Concatenation
Combine strings using the + operator or template literals:
Example:
let firstName = "John";
let lastName = "Doe";
let fullName = firstName + " " + lastName;
console.log(fullName); // Output: John Doe
- Or with template literals:
Example:
let fullNameTemplate = `${firstName} ${lastName}`;
console.log(fullNameTemplate); // Output: John Doe
String Length
Find the length of a string using .length:
Example:
let message = "Hello!";
console.log(message.length); // Output: 6
Accessing Characters
Access individual characters in a string using indexing or .charAt():
Example:
console.log(message[0]); // Output: H
console.log(message.charAt(1)); // Output: e
Useful String Methods
JavaScript provides several built-in methods to manipulate strings:
- 1. Convert to Uppercase:
Example:
let upperCase = message.toUpperCase(); // Output: HELLO!
- 2. Convert to Lowercase:
Example:
let lowerCase = message.toLowerCase(); // Output: hello!
- 3. Trim Whitespace:
Example:
let trimmed = " Hello! ".trim(); // Output: Hello!
- 4. Check for Substring:
Example:
let includesHello = message.includes("Hello"); // Output: true
Substring Extraction
Extract a portion of a string using .substring(start, end):
Example:
let substring = message.substring(1, 4);
console.log(substring); // Output: ell
Multi-Line Strings with Template Literals
Template literals allow for multi-line strings:
Example:
let multiLineString = `This is a string
that spans multiple
lines.`;
console.log(multiLineString);
String Replacement
Replace parts of a string using .replace():
Example:
let replacedString = message.replace("Hello", "Hi");
console.log(replacedString); // Output: Hi!
Conclusion
Strings are a vital tool for handling text in JavaScript. By mastering string methods, concatenation, and template literals, you can write more dynamic and efficient code. Experiment with the examples provided and explore how you can use strings to enhance your projects.