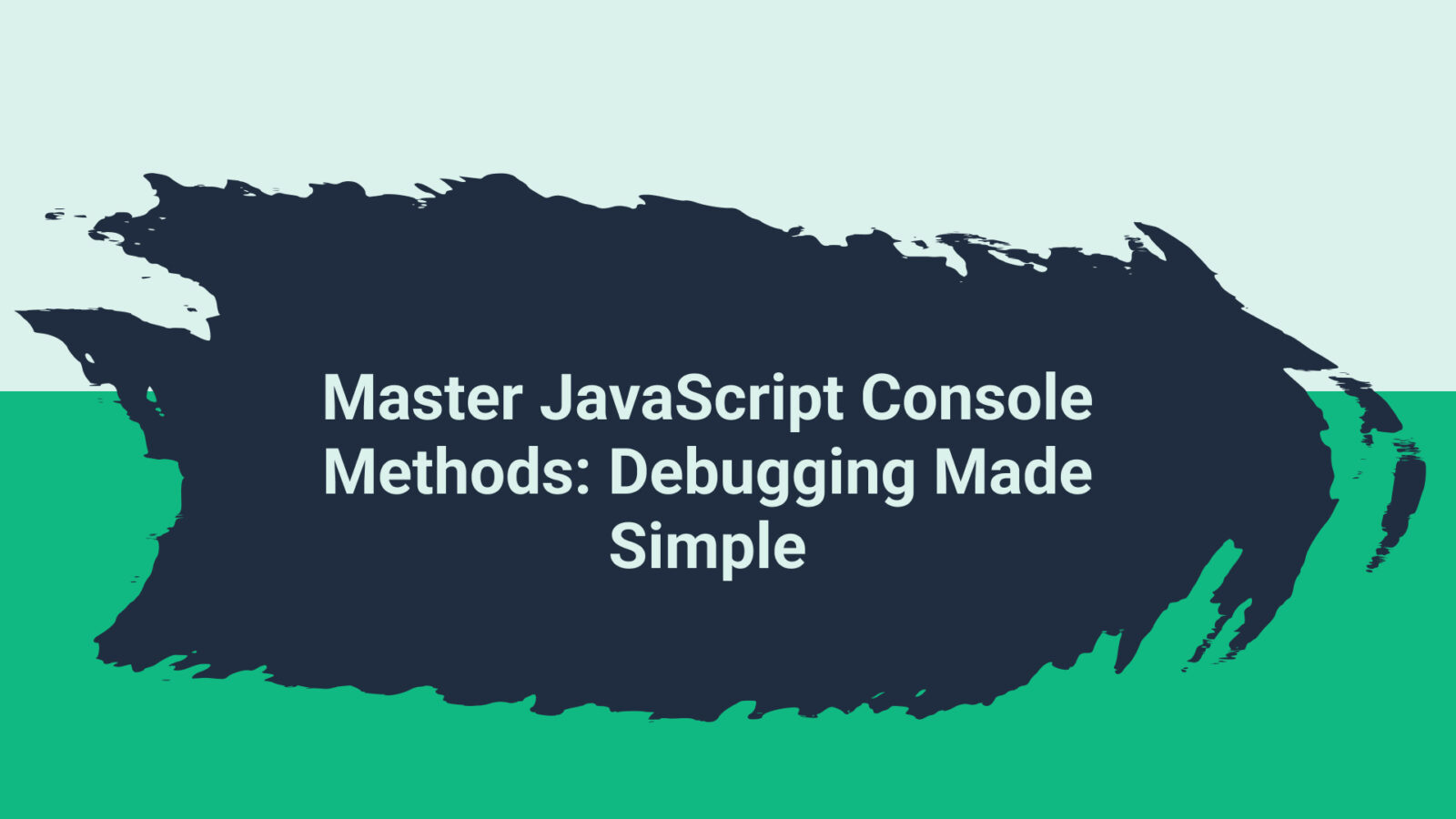
Master JavaScript Console Methods: Debugging Made Simple
Overview
Debugging is an essential part of software development, and JavaScript provides powerful console methods to help developers identify and fix issues efficiently. These methods, such as console.log(), console.error(), console.table(), and more, allow developers to monitor their code's behavior, analyze data, and understand how the application is functioning in real-time. This post dives into the most commonly used console methods, explaining their purpose and providing practical examples to simplify debugging and improve development workflow.
Here’s a simple, user-friendly explanation of each of these console methods in JavaScript:
1. console.log()
- What it does: Prints a regular message to the console.
- Use case: General-purpose messages.
Example:
console.log("Hello, World!");
2. console.error()
- What it does: Logs an error message to the console (often styled in red).
- Use case: Report problems or errors in your code.
Example:
console.error("Something went wrong!");
3. console.warn()
- What it does: Logs a warning message (usually styled in yellow).
- Use case: Highlight potential issues without stopping the program.
Example:
console.warn("This action is deprecated.");
4. console.info()
- What it does: Logs an informational message.
- Use case: Provide details or helpful information.
Example:
console.info("Server is running on port 3000.");
5. console.debug()
- What it does: Logs debugging information (hidden in some browsers by default).
- Use case: Useful for debugging during development.
Example:
console.debug("Debugging data: x = 42");
6. console.table()
- What it does: Displays data in a table format for easy readability.
- Use case: When working with arrays or objects.
Example:
console.table([{ name: "Alice", age: 25 }, { name: "Bob", age: 30 }]);
7. console.assert()
- What it does: Logs a message only if a condition is false.
- Use case: Test assumptions in your code.
Example:
console.assert(5 > 10, "This will log because the condition is false.");
8. console.time() & console.timeEnd()
- What they do: Measure how long a block of code takes to execute.
- Use case: Performance testing.
Example:
console.time("Loop Timer");
for (let i = 0; i < 1000000; i++) {}
console.timeEnd("Loop Timer"); // Logs the time taken
9. console.group() & console.groupEnd()
- What they do: Organize console logs into collapsible groups.
- Use case: Group related logs together.
Example:
console.group("My Group");
console.log("Inside the group");
console.groupEnd();
10. console.clear()
- What it does: Clears the console.
- Use case: Clean up the console during development.
Example:
console.clear();
Conclusion
Mastering JavaScript console methods is a crucial step in becoming a more efficient developer. These tools simplify debugging, help you visualize data, and allow you to track your application's behavior. Whether you’re a beginner or an experienced developer, leveraging these methods will streamline your workflow and improve the quality of your code. Start experimenting with these techniques today and see how they can transform your development process!