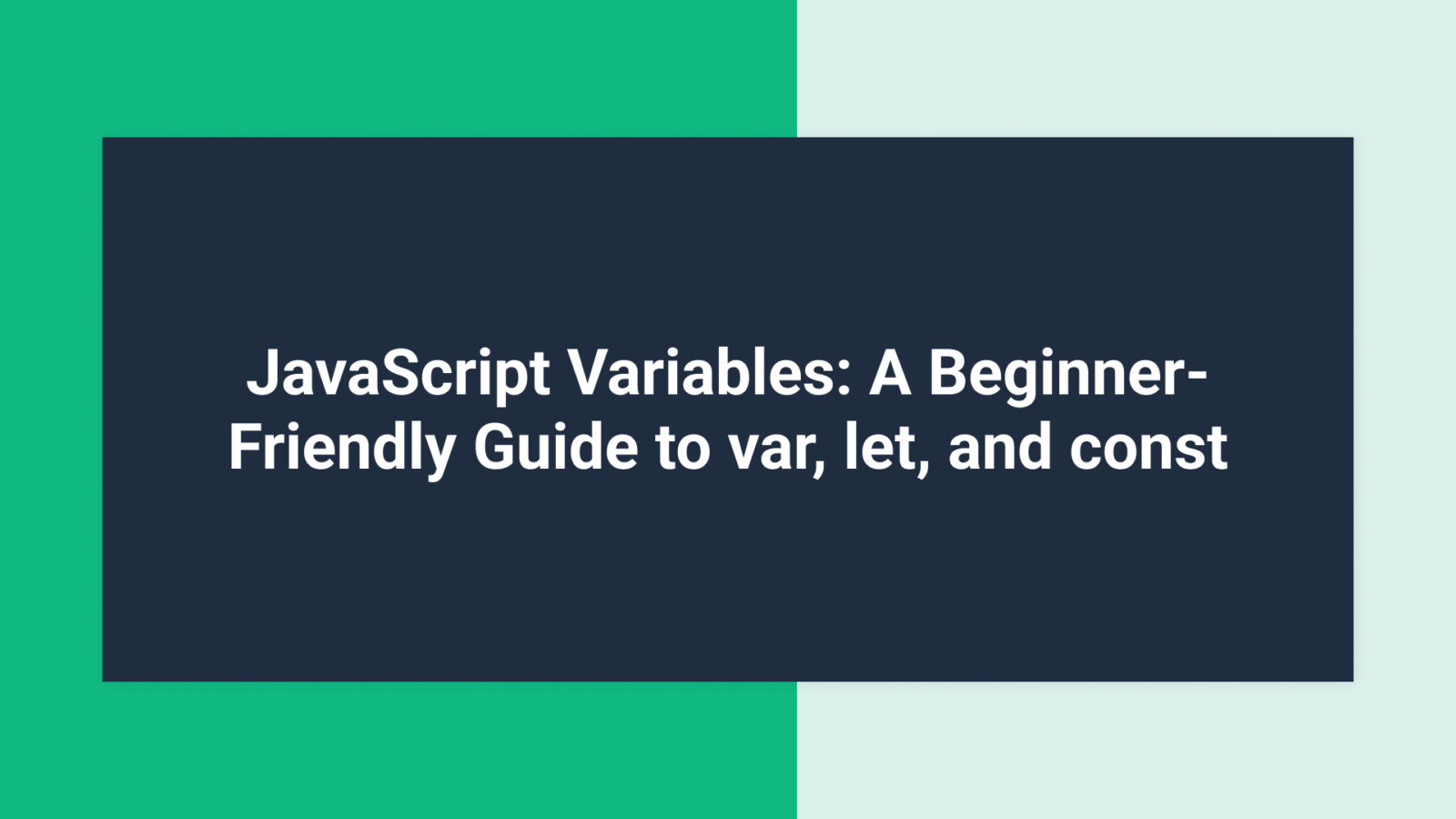
JavaScript Variables: A Beginner-Friendly Guide to var, let, and const
Overview
Understanding variables is fundamental in JavaScript programming. This guide simplifies the concept of var, let, and const, explaining their usage, scope, and when to choose one over the other. You'll also learn about dynamic typing, different data types, and how to use template literals with variables in JavaScript. Perfect for beginners who want clear examples and practical explanations.
Variable Declarations in JavaScript
- Using var:
Variables declared with var are either function-scoped or globally-scoped, meaning their accessibility depends on where they are declared.
Example:
var name = "Rapidcheat.Com";
console.log(name); // Output: Rapidcheat.Com
- Using let:
Variables declared with let are block-scoped, meaning they are only accessible within the block they are defined in.
Example:
let age = 25;
console.log(age); // Output: 25
- Using const:
Variables declared with const are also block-scoped but cannot be reassigned after their initial declaration.
Example:
const pi = 3.14;
console.log(pi); // Output: 3.14
Reassigning Variables
- Allowed with let:
You can reassign variables declared with let.
Example:
age = 26;
console.log(age); // Output: 26
- Not Allowed with const:
Variables declared with const cannot be reassigned.
Variable Types in JavaScript
JavaScript supports multiple data types, including:
- Boolean: Represents true or false.
Example:
let isActive = true;
- Null: Represents an empty value.
Example:
let score = null;
- Undefined: A variable declared but not assigned a value.
Example:
let user;
Dynamic Typing
JavaScript is dynamically typed, meaning a variable's type can change during execution.
Example:
let dynamicVar = 10; // Initially a number
dynamicVar = "Hello"; // Now a string
dynamicVar = [1, 2, 3]; // Now an array
Template Literals
Template literals allow you to include variables directly within strings using ${}.
Example:
let greeting = `Hello, ${name}! You are ${age} years old.`;
console.log(greeting); // Output: Hello, Rapidcheat.Com! You are 26 years old.
Conclusion
Understanding how to use var, let, and const is essential for writing effective JavaScript code. By choosing the right declaration method and knowing when reassignment is possible, you'll write cleaner, more predictable programs. Experiment with the examples provided and see how they apply to your projects.